How to Reverse a List in Python (Using 3 Simple Ways)
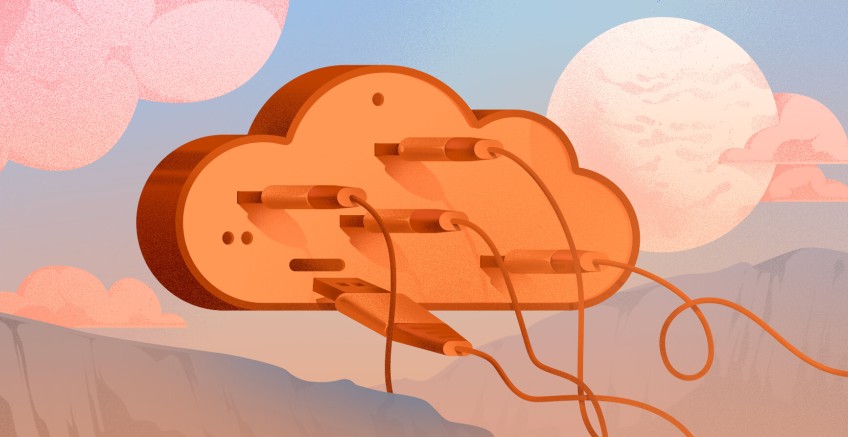
A list is a data structure used to manipulate and store ordered groupings of objects. This is thanks to their ability to hold various data types, including numbers and strings. They support dynamic resizing and it makes them perfect for handling varying amounts of data. Common operations on lists include appending elements, slicing, sorting, and reversing, which are essential for data processing tasks. In algorithms, reversing a list is a beneficial operation when the order of the components needs to be inverted.
This article will cover
- Various methods to reverse a list in Python
- How each method performs in terms of time and memory usage.
- Common mistakes to watch out for when using these methods.
#What does it mean to reverse a list in Python?
Reversing a list means arranging its items in the opposite order from how they started. Basically, the first item will be moved to the last position, the second item to the second-to-last, and this pattern continues for the entire list. The need to perform this operation typically arises from scenarios where data ordering is important. For example, working with time-based data that are to be analyzed in an inverse chronological order or reversing the order of steps in a process. If you, for example, have a list of tasks where you have captured them from beginning to end, reversing this list will give you tasks from end to start.
However, it is important to remember that reversing is not the same as sorting.
Reversing alters the arrangement of elements concerning how they were listed while sorting rearranges them based on a given criterion and orders them afresh.
Deploy and scale your Python projects effortlessly on Cherry Servers' robust and cost-effective dedicated or virtual servers. Benefit from an open cloud ecosystem with seamless API & integrations and a Python library.
#Basic methods to reverse a list in Python
To reverse a list in Python, two basic methods are commonly used, the built-in reverse()
method and slicing with [::-1].
The reverse()
method directly reverses the elements of the list in place. It updates the existing list without generating another one. This method saves memory but changes the original list. It is efficient when you don’t need to keep the original order.
orginalList = [1, 2, 3, 4, 5]
orginalList.reverse()
print(orginalList)
Output:
On the other hand, slicing with [::-1] creates a new list that is a reversed copy of the original. This approach maintains the original data but requires extra memory to store the reversed list.
orginalList = [1, 2, 3, 4, 5]
reversedList = orginalList[::-1]
print(reversedList)
Output:
Each method has its advantages depending on whether you need to keep the original list unchanged.
#Reversing a list in-place vs. creating a new list
The reversal of a list in-place requires modifying the original list directly, rather than creating a completely new one.This is done using the reverse()
method in Python. When you call reverse()
on a list, it reorders the elements in reverse within the same memory space.
If you need to reverse a list without altering the original, you can use slicing with [::-1]. This method allows you to create a new list which is the reverse of the original list. In this method, the original list is not changed.
Keep your requirements in mind when choosing between the 2 options discussed above. Opt for in-place reversal if memory efficiency is important and you don’t need to retain the original list order. Alternatively, choose to create a new reversed list when preserving the original data is important and you have enough memory to handle the additional list. This approach is particularly useful when the original list will be referenced or compared later.
#Reversing lists with a loop
You can manually reverse a list by creating a new list with all of the components from the original list added in reverse order. This approach mixes Python syntax with the logic of a loop.
Loop-based reversal follows a simple logic. Simply begin at the last item in the list and work your way backwards while collecting each element to create a new list.
orginalList = [1, 2, 3, 4, 5]
reversedList = [orginalList[i] for i in range(len(orginalList) - 1, -1, -1)]
print(reversedList)
Output:
In the above example, range(len(my_list) - 1, -1, -1)
generates indices from the last element to the first, and the list comprehension iterates over these indices to build the reversed list.
This method gives flexibility in customizing the reversal process. It is useful when more complex operations need to be performed during the reversal.
#Using the reversed() Function
Python’s reversed()
function offers an easy method to reverse the elements of a list. Unlike other methods, reversed()
doesn't make a new or changed list directly. Instead, it returns an iterator that can be used to go through the list in reverse order. This approach is efficient because it avoids creating a new list immediately. Therefore it is useful when working with large datasets.
orginalList = [1, 2, 3, 4, 5]
reversedIterator = reversed(orginalList)
print(reversedIterator)
Output:
The reversed()
function returns an iterator, which means you can loop through it to access the elements in reverse. However, if you need a reversed list, you can easily convert this iterator into a list using the list()
function.
reversedList = list(reversed(orginalList))
print(reversedList)
Output:
This method is useful when you want to iterate over a list in reverse without creating a copy unless explicitly needed.
Deploy and scale your projects with Cherry Servers' cost-effective dedicated or virtual servers. Enjoy seamless scaling, pay-as-you-go pricing, and 24/7 expert support—all within a hassle-free cloud environment.
#Performance considerations
When evaluating the performance of different list reversal methods in Python, 2 aspects need to be considered. They are discussed below.
#Time complexity
This measures how the execution time of an algorithm increases with the size of the input list. For reversing a list, all methods discussed have a time complexity of O(n), where n is the number of elements in the list. This means they all require a linear amount of time relative to the list size.
#Auxiliary space
This refers to the additional memory used by the algorithm.
-
reverse()
Method: The space complexity is O(1), which is optimal for memory usage. This is because it doesn't require extra space beyond what’s needed for the original list. -
Slicing with [::-1]: Its auxiliary space complexity is O(n) because it requires additional memory proportional to the size of the input list.
-
Loop-Based Reversal: Similar to slicing, if implemented to create a new list, it also has an auxiliary space complexity of O(n). If the reversal is done in place within the list, it uses O(1) space.
-
reversed()
Function: This returns an iterator, which is memory-efficient with O(1) auxiliary space until converted to a list. However, if you want to convert the iterator to a list, that would result in O(n) space complexity.
For large lists, prefer using reverse()
or reversed()
to conserve memory. Slicing with [::-1] is suitable for small to moderate-sized lists where readability is important, despite its higher memory usage. Opt for loop-based reversal if you need to perform custom operations during the reversal process.
#Common pitfalls and how to avoid them
It may be rather challenging to reverse a list in Python because of the following issues.
#Mistakes to avoid
Using the reverse()
method modifies the original list. Make sure that the modified list is acceptable for your use case. If you need to keep the original list unchanged, consider using slicing ([::-1]) or the reversed()
function instead. Additionally, ensure that all elements are compatible with the reversal method, as attempting to reverse an iterator directly without first converting it to a list can result in errors if not managed correctly.
#Issues with nested lists or mixed data types
Here we will discuss issues related to nested lists and mixed data types.
-
Nested Lists: Reversal methods apply to the list’s top level. For nested lists, you might need to individually reverse each sublist if necessary. The methods discussed will reverse the top-level list but not elements within nested lists.
-
Mixed Data Types: While reversing a list with mixed data types is generally supported, make sure that your data types are compatible with the operations you intend to perform after reversal.
#Troubleshooting common errors
Here are some common errors and some tips on how to troubleshoot them.
-
Attribute Errors: Check twice whether you are applying the right method for the data type. For instance, calling
reverse()
on a non-list object will result in an AttributeError. -
Index Errors: Verify that slicing or looping does not go out of bounds. Ensure indices are within the valid range of the list.
-
Type Errors with
reversed():
Keep in mind thatreversed()
returns an iterator. If you want to access the reversed items directly, convert it to a list.
#Practical applications of reversing a list
Reversing a list proves valuable in numerous real-world situations. In data processing, reversing a list can help analyze time-based data in reverse chronological order, such as sorting logs from most recent to oldest. Algorithms often use list reversal to solve problems like checking for palindromes or reversing the order of operations. For example, in algorithms like depth-first search, reversing the adjacency list can help with traversing nodes efficiently. Reversing a list can also be practical in user interfaces, such as displaying the most recent notifications at the top.
Also read: How to install pandas in python
#Conclusion
Reversing a list in Python is an important operation with multiple applications across data processing, algorithms, and user interfaces. Each approach discussed above offers unique advantages depending on your needs. Understanding when to use in-place reversal versus creating a new list is essential for efficient memory usage. Additionally, manual reversal using loops or comprehensions provides flexibility for custom operations. By recognizing common pitfalls and performance considerations, you can easily choose the right method for your tasks and manipulate lists efficiently in your Python projects.
Found this guide helpful? read our Python tutorials on how to manage Conda environments, how to use a Boolean in Python and how to install PyTorch on Ubuntu.
Cloud VPS Hosting
Starting at just $3.24 / month, get virtual servers with top-tier performance.