How to Convert String to Float in Python (6 Different Ways)
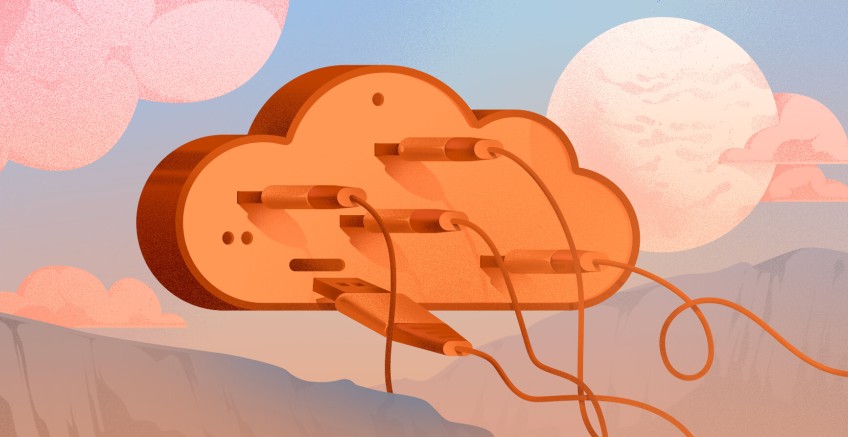
Converting values in a string data type into float is a common task in Python. It is especially useful when handling user input or data from external sources. It enables users to perform mathematical operations on numeric values that were initially in the string format.
#Converting String to Float
There are a few methods to convert string to float in Python. However, we will focus only on the following methods within this article.
- Using the
float()
function. - Using the
Decimal()
function. - Using the ‘ast’ Module for Literal Evaluation.
- Convert string to float using Numpy.
- Using the
json.loads()
. - Using a custom function.
Now let’s go through each of these methods in more detail.
Deploy and scale your Python projects effortlessly on Cherry Servers' robust and cost-effective dedicated or virtual servers. Benefit from an open cloud ecosystem with seamless API & integrations and a Python library.
#Using the float() function
Using the float()
function is the most common way to convert string to float in Python. What’s more, it is a built-in function which means it comes with standard Python installation. You can pass a string value as the argument to it and get the corresponding floating-point number. Have a look at the following example to gain a more clear idea of this.
string_number = "13.86"
float_number = float(string_number)
print(float_number)
print(type(float_number))
Output:
As you can see, the float function returns a value of the <class 'float'>
. However, the function will return a ValueError if the input value passed into it doesn't represent a valid floating-point number. For example, look at the following code block.
string_number = "invalid float"
float_number = float(string_number)
print(float_number)
print(type(float_number))
Output:
Therefore, it's important to use proper error-handling mechanisms like try-except blocks while coding. This approach is very useful, especially when converting user inputs as you can't predict what kind of input a user will insert. The following example shows how to use the float()
function for user inputs at its best.
user_input = input("Enter a number: ")
try:
float_number = float(user_input)
print("Valid float:", float_number)
except ValueError:
print("This is not a valid floating-point number.")
#Using the Decimal() function
In Python, for scenarios requiring high precision and accuracy in floating-point arithmetic, the Decimal()
function from the decimal module can be used. The important thing about the Decimal()
function is that it’s more precise compared to the built-in float()
function, which can sometimes introduce a small error due to the binary floating-point representation. This function takes a string or a number as an argument and returns a Decimal object representing the exact decimal number specified.
from decimal import Decimal
string_number = "13.86"
decimal_number = Decimal(string_number)
print(decimal_number)
print(type(decimal_number))
Output:
As shown in the above image, the Decimal() function returns a value of the <class 'decimal.Decimal'>
. If the input value passed to Decimal() does not represent a valid number, it will raise a ValueError or InvalidOperation, similar to the float()
function.
The Decimal()
function is particularly useful over float()
when you need more precision in decimal arithmetic, such as in financial calculations, or scientific computing.
#Using the ‘ast’ Module for Literal Evaluation
Another method to convert a string to a float is by using the ast (Abstract Syntax Trees) module. The primary purpose of the ast.literal_eval()
function is to evaluate strings containing Python literals. However, it can also be used to convert strings to floats, provided the strings passed to it contain only a floating-point number. In such cases, this method returns the corresponding Python floating-point object. Let’s see an example of the ast.literal_eval()
function in action.
import ast
input_string = "33.3"
float_number = ast.literal_eval(input_string)
print(float_number)
print(type(float_number))
Output:
As shown in the above example, the ast.literal_eval()
function evaluates the string "33.3" as a float, yielding the float data type.
#Convert string to float with Numpy
As a Python developer, you may have already heard about NumPy. It is a popular Python library for scientific computing. It is widely used for advanced mathematical operations, especially when dealing with arrays and matrices. If you're planning to use NumPy for your next project, it is essential to know how to convert strings to floats with this library.
Numpy includes a data type called numpy.float64, which represents double-precision floating-point numbers. You can also use this data type to convert string values into floating-point numbers. Here is an example of how to do that.
import numpy as np
my_value = "64.9512"
float_number = np.float64(my_value)
print(float_number)
print(type(float_number))
Output:
This code snippet converts the string "64.9512" to a NumPy float64. NumPy is particularly useful for handling arrays of values, making it an excellent choice for converting multiple strings to floats in the context of numerical computing.
Similar to the float() function, using numpy.float64 to convert a string that does not represent a valid floating-point number will result in a ValueError.
#Using the json.loads()
This is another simplest way to convert string to float in Python. However, let’s look into it as well for the purpose of this tutorial. This method comes in handy when dealing with JSON data, which is very common in API interactions.
This json.loads()
function of the Python JSON module is essential when working with data in JSON format. It offers a significant advantage by allowing users to convert a JSON-formatted string into a Python dictionary. It also facilitates easy data manipulation.
When you pass JSON values containing floating-point numbers, json.loads()
will automatically convert them to the float data type. Here is an example.
import json
json_string = '{"temperature": 23.5}'
python_data = json.loads(json_string)
temperature = python_data['temperature']
print(temperature)
print(type(temperature))
Output:
This example shows how json.loads()
parses a JSON string, converting the numerical value "23.5" from the string type into a float. This function will raise a json.JSONDecodeError if the JSON string has an incorrect structure or contains improperly formatted numerical values.
Also read: How to manage Conda environments
#Using a custom function
Sometimes, you might come across situations where using built-in functions like float()
is not appropriate for converting string to float. In such cases, you can gain the required flexibility by creating a custom function to achieve the same purpose. Although this method involves a bit more work, it allows for specific error handling or conversion logic that standard functions lack.
Let’s take a look at an example code piece of implementing custom logic to convert string to float.
def string_to_float(string):
number = 0.0
# Check if the string represents a negative number
is_negative = string[0] == '-'
# Find the position of the decimal point
decimal_position = string.find('.')
# If there is no decimal point, treat it as an integer part
if decimal_position == -1:
decimal_position = len(string)
# Convert the integer part
for char in string[:decimal_position]:
if char.isdigit():
number = number * 10 + (ord(char) - ord('0'))
# Convert the fractional part
fractional_part = 0.1
for char in string[decimal_position+1:]:
if char.isdigit():
number += (ord(char) - ord('0')) * fractional_part
fractional_part *= 0.1
# Apply negative sign if needed
return -number if is_negative else number
# Example usage
string_number = "123.45"
converted_number = string_to_float(string_number)
print(converted_number)
print(type(converted_number))
Output:
The above code is very self-explanatory with the relevant comments for specific code blocks. If you are a Python developer, it’s essential to know how to write a custom function to convert string to float. In some cases, you will have to deal with numerical data embedded within text files. These files might have a non-standard delimiter for the decimal point, such as a comma (',')
instead of a period ('.')
. This situation is very common when working with various locale systems different from English-based locales. In such instances, you can develop a custom function to interpret these formats correctly.
#Conclusion
Converting string to float is a typical task that any programmer has to go through. This article described six different ways to convert a string to a float. Each method varies in terms of efficiency, security, and customizability. Here, we also discussed when to use each method based on different criteria. By practicing these methods, you will be able to apply them in even more complex scenarios that you will encounter in your development journey. Happy coding!
Cloud VPS Hosting
Starting at just $3.24 / month, get virtual servers with top-tier performance.